共计 793 个字符,预计需要花费 2 分钟才能阅读完成。
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).
For example, this binary tree [1,2,2,3,4,4,3]
is symmetric:
1
/ \
2 2
/ \ / \
3 4 4 3
But the following [1,2,2,null,3,null,3]
is not:
1
/ \
2 2
\ \
3 3
Note:
Bonus points if you could solve it both recursively and iteratively.
解法
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def isSymmetric(self, root: TreeNode) -> bool:
if not root:
return True
dq = collections.deque([(root.left,root.right),])
while dq:
node1, node2 = dq.popleft()
#两个都是空节点直接跳过
if not node1 and not node2:
continue
#只要其中一个节点为空直接返回False
if not node1 or not node2:
return False
#若两个节点都存在,那么需要判断两者的值是否相等
if node1.val != node2.val:
return False
# 判断左节点的左节点与右节点的右节点
# 判断左节点的右节点和右节点的左节点
dq.append((node1.left,node2.right))
dq.append((node1.right,node2.left))
return True
正文完
请博主喝杯咖啡吧!
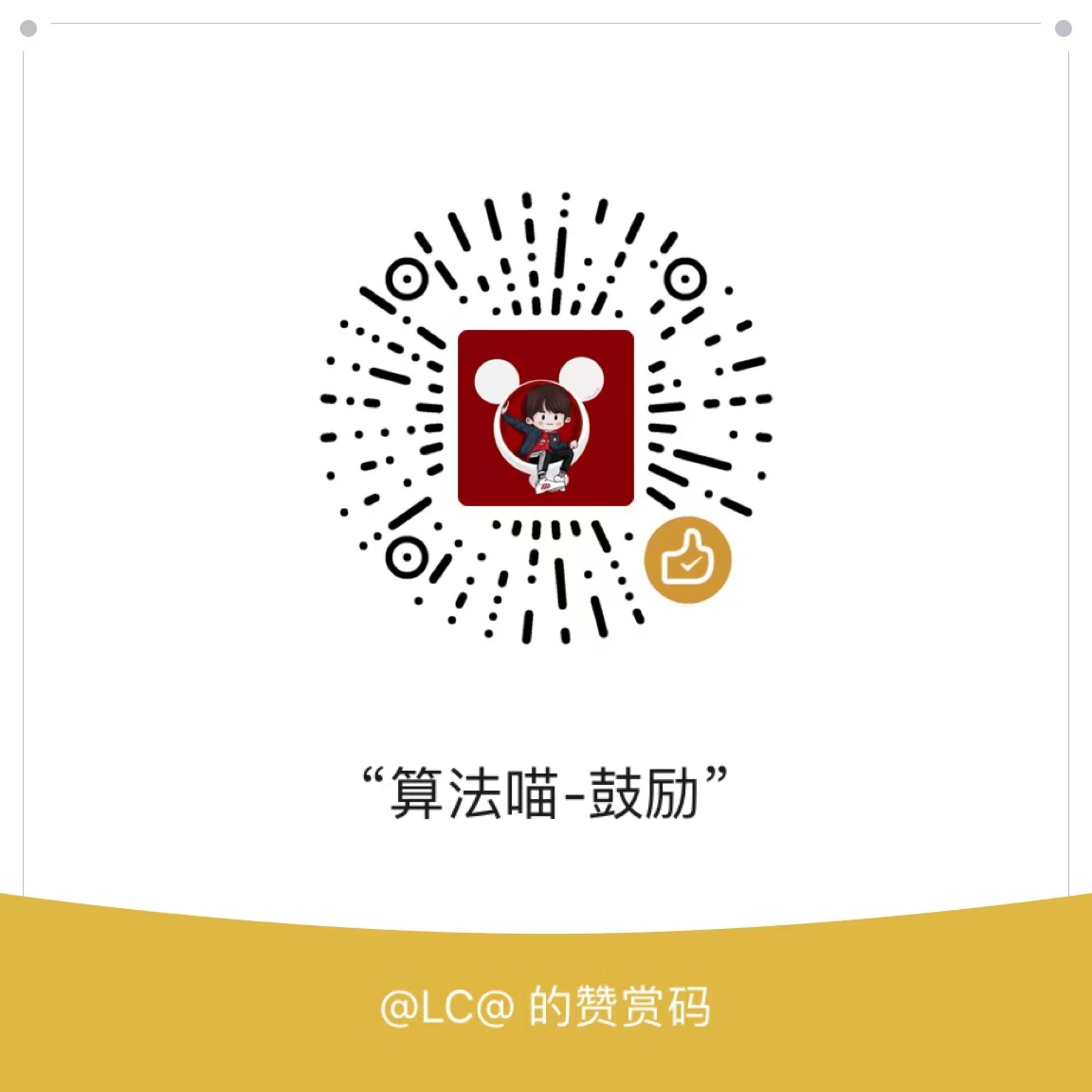