共计 4336 个字符,预计需要花费 11 分钟才能阅读完成。
qt提供了三种方式解析xml,不过如果想实现对xml文件进行增、删、改等操作,还是DOM方式最方便。
QT += core xml
QT -= gui
TARGET = xmltest
CONFIG += console
CONFIG -= app_bundle
TEMPLATE = app
SOURCES += main.cpp
#include <QCoreApplication>
#include<QDomComment>
#include<QFile>
#include<QTextStream>
#include<QDebug>
//写操作
void Dom_xml_write()
{
QFile file("dom_test.xml");
if(!file.open(QFile::WriteOnly| QFile::Truncate))
{
return;
}
// XML declaration
QDomDocument doc;
QDomProcessingInstruction instuct;
instuct=doc.createProcessingInstruction("xml","version=\"1.0\" encoding=\"UTF-8\"");
doc.appendChild(instuct);
//创建节点
QDomElement root=doc.createElement("deeplearn");
doc.appendChild(root);
//创建子节点
QDomElement qt_channel=doc.createElement("qt_channel");
qt_channel.setAttribute("id",1);
QDomAttr qt_start_time=doc.createAttribute("time");
qt_start_time.setValue("2015/05/01");
qt_channel.setAttributeNode(qt_start_time);
QDomElement qt_title=doc.createElement("qt_title");
QDomText qt_text=doc.createTextNode("qt");
qt_title.appendChild(qt_text);
qt_channel.appendChild(qt_title);
root.appendChild(qt_channel);
//创建子节点
QDomElement ml_channel=doc.createElement("ml_channel");
ml_channel.setAttribute("id",2);
QDomAttr ml_start_time=doc.createAttribute("time");
ml_start_time.setValue("2015/05/02");
ml_channel.setAttributeNode(ml_start_time);
QDomElement ml_title=doc.createElement("ml_title");
QDomText ml_text=doc.createTextNode("ml");
ml_title.appendChild(ml_text);
ml_channel.appendChild(ml_title);
root.appendChild(ml_channel);
QTextStream filestream(&file);
doc.save(filestream,4);
file.close();
}
//读操作
void Dom_xml_read()
{
QFile file("dom_test.xml");
if(!file.open(QFile::ReadOnly))
{
return;
}
QDomDocument doc;
if(!doc.setContent(&file))
{
file.close();
return;
}
file.close();
//获取根节点
QDomElement root=doc.documentElement();
qDebug()<<root.nodeName();
QDomNode first=root.firstChild();
while(!first.isNull())
{
if(first.isElement())
{
QDomElement e=first.toElement(); //转换为元素,注意元素和节点是两个数据结构,其实差不多
qDebug()<<e.tagName()<<" "<<e.attribute("id")<<" "<<e.attribute("time"); //打印键值对,tagName和
// Name是一个东西
QDomNodeList list=e.childNodes();
for(int i=0;i<list.count();i++) //遍历子元素,count和size都可以用,可用于标签数计数
{
QDomNode n=list.at(i);
if(first.isElement())
qDebug()<<n.nodeName()<<":"<<n.toElement().text();
}
}
first=first.nextSibling(); //下一个兄弟节点,nextSiblingElement()是下一个兄弟元素,都差不多
}
}
//修改xml
void Dom_xml_add()
{
QFile file("dom_test.xml");
if(!file.open(QFile::ReadOnly))
{
return;
}
QDomDocument doc;
if(!doc.setContent(&file))
{
file.close();
return;
}
file.close();
//创建节点
QDomElement root=doc.documentElement();
//创建子节点
QDomElement qt_channel=doc.createElement("qt_channel");
qt_channel.setAttribute("id",1);
QDomAttr qt_start_time=doc.createAttribute("time");
qt_start_time.setValue("2015/05/01");
qt_channel.setAttributeNode(qt_start_time);
QDomElement qt_title=doc.createElement("qt_title");
QDomText qt_text=doc.createTextNode("qt");
qt_title.appendChild(qt_text);
qt_channel.appendChild(qt_title);
root.appendChild(qt_channel);
if(!file.open(QFile::WriteOnly | QFile::Truncate))
{
file.close();
return;
}
QTextStream out(&file);
doc.save(out,4);
file.close();
}
void Dom_xml_delete()
{
QFile file("dom_test.xml");
if(!file.open(QFile::ReadOnly))
{
return;
}
QDomDocument doc;
if(!doc.setContent(&file))
{
file.close();
return;
}
file.close();
//获取根节点
QDomElement root=doc.documentElement();
QDomNodeList curr_list=doc.elementsByTagName("qt_channel");
for(int i=0;i<curr_list.size();i++)
{
if(curr_list.at(i).isElement())
{
QDomElement e=curr_list.at(i).toElement();
if(e.attribute("time")=="2015/05/01") //以属性名定位,类似于hash的方式
{
//删除根节点的子节点
root.removeChild(curr_list.at(i));
}
}
}
if(!file.open(QFile::WriteOnly | QFile::Truncate))
{
file.close();
return;
}
QTextStream out(&file);
doc.save(out,4);
file.close();
}
void Dom_xml_update()
{
QFile file("dom_test.xml");
if(!file.open(QFile::ReadOnly))
{
return;
}
QDomDocument doc;
if(!doc.setContent(&file))
{
file.close();
return;
}
file.close();
//获取根节点
QDomElement root=doc.documentElement();
QDomNodeList curr_list=doc.elementsByTagName("qt_channel");
//获取第一个子节点信息
QDomNode node=curr_list.at(0).firstChild();
QDomNode old_node=node.firstChild();
node.firstChild().setNodeValue("my_qt");
QDomNode new_node=node.firstChild();
node.replaceChild(new_node,old_node);
if(!file.open(QFile::WriteOnly | QFile::Truncate))
{
file.close();
return;
}
QTextStream out(&file);
doc.save(out,4);
file.close();
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
//Dom_xml_write();
// Dom_xml_read();
//Dom_xml_add();
//Dom_xml_delete();
Dom_xml_update();
return a.exec();
}
实现的结果如下:
初始写xml:
xml增加:
xml删除:
xml更新:
正文完
请博主喝杯咖啡吧!
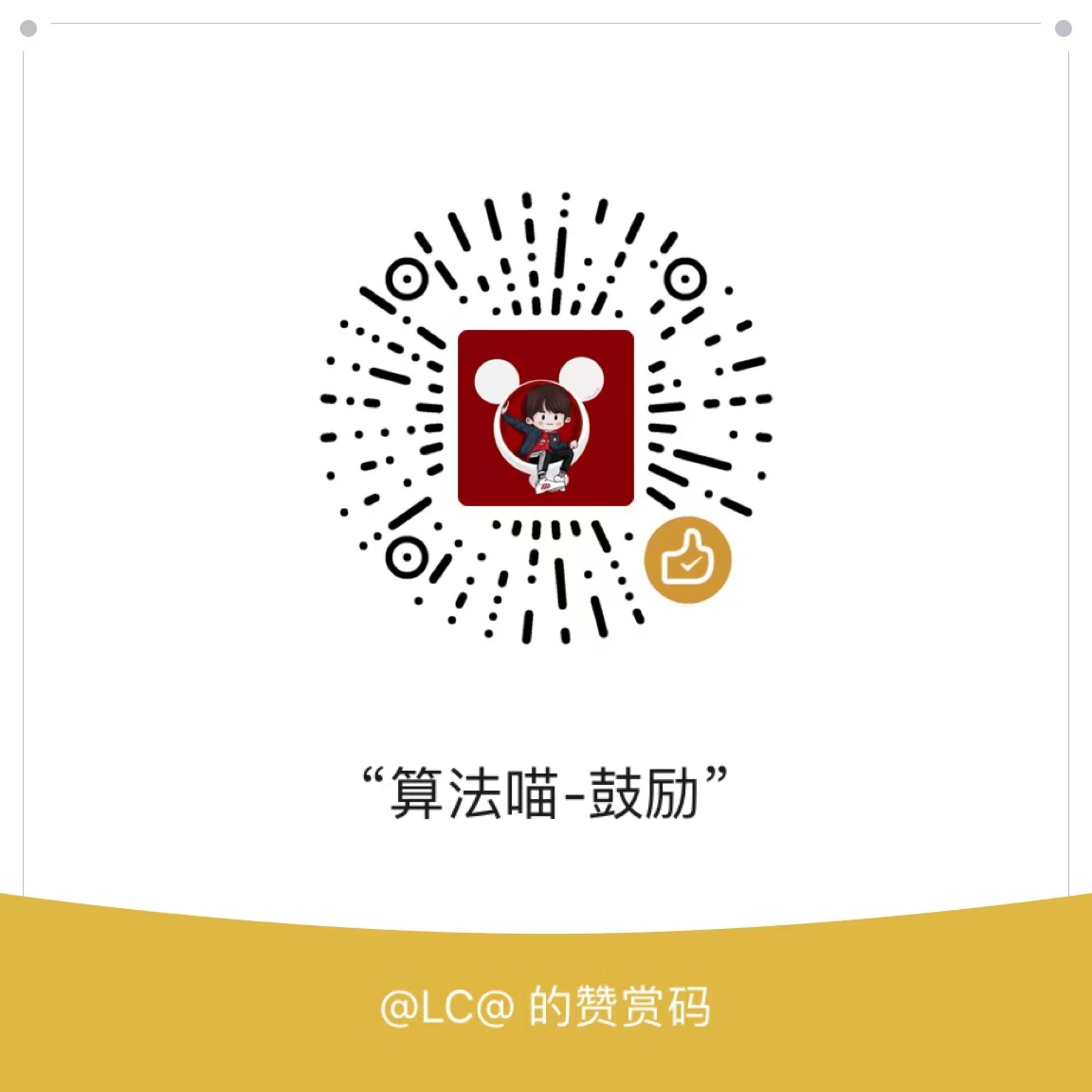