共计 4384 个字符,预计需要花费 11 分钟才能阅读完成。
The term broadcasting describes how numpy treats arrays with different shapes during arithmetic operations. Subjectto certain constraints, the smaller array is “broadcast” across the larger array so that they have compatible shapes.Broadcasting provides a means of vectorizing array operations so that looping occurs in C instead of Python. It doesthis without making needless copies of data and usually leads to efficient algorithm implementations. There are,however, cases where broadcasting is a bad idea because it leads to inefficient use of memory that slows computation.
广播机制是为了不同大小的矩阵活着数组之间运算时使用,最小的数组会通过的广播的形式扩展到维数较大的数组一致。广播的机制对内存的利用效率较差会降低计算的速度
NumPy operations are usually done on pairs of arrays on an element-by-element basis. In the simplest case, the twoarrays must have exactly the same shape, as in the following example:
numpy的运算经常在成对的数组上运算,以元素一一对应的方式,举个简单的例子就是两个相同大小的数组相加运算
NumPy’s broadcasting rule relaxes this constraint when the arrays’ shapes meet certain constraints. The simplestbroadcasting example occurs when an array and a scalar value are combined in an operation:
The result is equivalent to the previous example where b was an array. We can think of the scalar b being stretchedduring the arithmetic operation into an array with the same shape as a. The new elements in b are simply copiesof the original scalar. The stretching analogy is only conceptual. NumPy is smart enough to use the original scalarvalue without actually making copies, so that broadcasting operations are as memory and computationally efficient aspossible.
The code in the second example is more efficient than that in the first because broadcasting moves less memory aroundduring the multiplication (b is a scalar rather than an array).
>>> a = np.array([1.0, 2.0, 3.0])
>>> b = np.array([2.0, 2.0, 2.0])
>>> a * b
array([ 2., 4., 6.])
>>> a = np.array([1.0, 2.0, 3.0])
>>> b = 2.0
>>> a * b
array([ 2., 4., 6.])
When operating on two arrays, NumPy compares their shapes element-wise. It starts with the trailing dimensions, andworks its way forward. Two dimensions are compatible when
1. they are equal, or
2. oneofthemis1
当对两个数组运算时,先比较它们的大小,从最后一维开始依次向前,只有满足以下条件才可以进行广播运算:
1、二者的形状大小一致
2、其中有一个维度大小为1
If these conditions are not met, a ValueError: frames are not aligned exception is thrown, indicatingthat the arrays have incompatible shapes. The size of the resulting array is the maximum size along each dimension ofthe input arrays.
如果这些条件不满足会出现ValueeError
Arrays do not need to have the same number of dimensions. For example, if you have a 256x256x3 array of RGBvalues, and you want to scale each color in the image by a different value, you can multiply the image by a one-dimensional array with 3 values. Lining up the sizes of the trailing axes of these arrays according to the broadcastrules, shows that they are compatible:
When either of the dimensions compared is one, the other is used. In other words, dimensions with size 1 are stretchedor “copied” to match the other.
In the following example, both the A and B arrays have axes with length one that are expanded to a larger size duringthe broadcast operation:
Here are some more examples:
Image (3d array): 256 x 256 x 3
Scale (1d array): 3
Result (3d array): 256 x 256 x 3
————————————–
A (4darray): 8x1x6x1
B (3d array):7 x 1 x 5
Result(4darray): 8x7x6x5
——————————————————
A (2d array): 5x 4
B (1d array): 1
Result (2d array): 5 x 4
————————————–
A (2d array): 5x 4
B (1d array): 4
Result (2d array): 5 x 4
————————————–
A (3d array): 15x3x5
B (3d array): 15x1x5
Result (3d array): 15 x 3 x 5
————————————–
A (3d array): 15x3x5
B (2d array): 3×5
Result (3d array): 15 x 3 x 5
————————————–
A (3d array): 15x3x5
B (2d array): 3×1
Result (3d array): 15 x 3 x 5
下面给出几个例子是不可以使用广播
An example of broadcasting in practice:
A (1d array): 3
B (1d array): 4 # trailing dimensions do not match
————————————–
A (2d array): 2 x 1
B (3d array): 8 x 4 x 3 # second from last dimensions mismatched
下面举个例子
>>> x = np.arange(4)
>>> xx = x.reshape(4,1)
>>> y = np.ones(5)
>>> z = np.ones((3,4))
>>> x.shape(4,)
>>> y.shape(5,)
>>> x + y
<type ‘exceptions.ValueError’>: shape mismatch: objects cannot be broadcast to a single
>>> xx.shape(4, 1)
>>> y.shape(5,)
>>> (xx + y).shape(4, 5)
>>> xx + y
array([[ 1., 1., 1., 1., 1.],
[ 2., 2., 2., 2., 2.],
[ 3., 3., 3., 3., 3.],
[ 4., 4., 4., 4., 4.]])
>>> x.shape
(4,)
>>> z.shape
(3, 4)
>>> (x + z).shape
(3, 4)
>>> x + z
array([[ 1., 2., 3., 4.],
[ 1., 2., 3., 4.],
[ 1., 2., 3., 4.]])
Broadcasting provides a convenient way of taking the outer product (or any other outer operation) of two arrays. Thefollowing example shows an outer addition operation of two 1-d arrays:
在之前的例子中给出反例:两个一维数组是无法进行广播运算,现在可以通过对其中一个一维数组添加额外一维,这样构造满足符合广播运算的条件,下面给出实际的例子:
>>> a = np.array([0.0, 10.0, 20.0, 30.0])
>>> b = np.array([1.0, 2.0, 3.0])
>>> a[:, np.newaxis] + b
array([[ 1., 2., 3.],
[ 11., 12., 13.],
[ 21., 22., 23.],
[ 31., 32., 33.]])
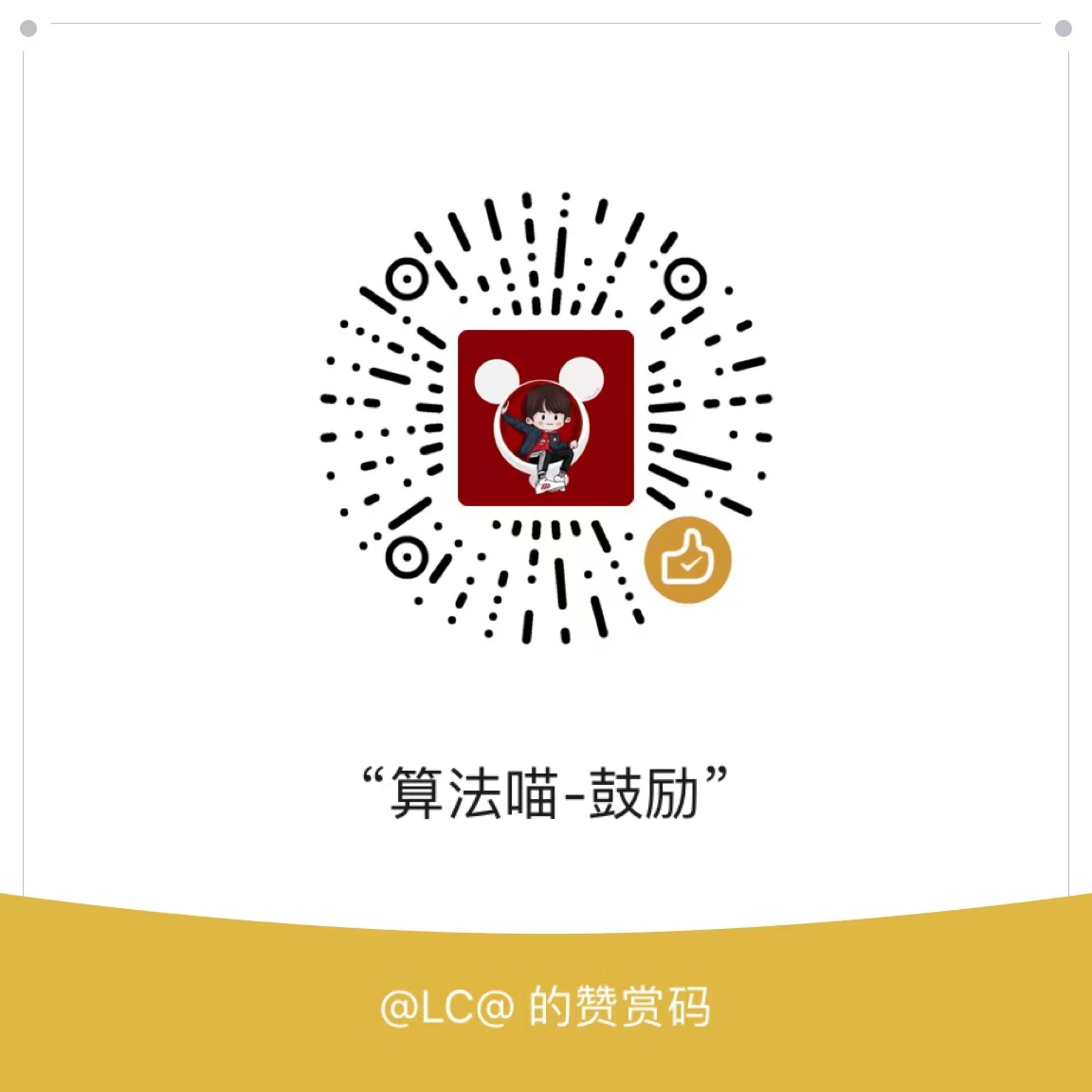